Welcome to the intricate world of medical device surveillance, a place where data isn't just numbers but a story waiting to be told. In this detective story, our tools are Python, machine learning algorithms, and curiosity. Let's embark on this journey to unravel the mysteries hidden within mountains of data.
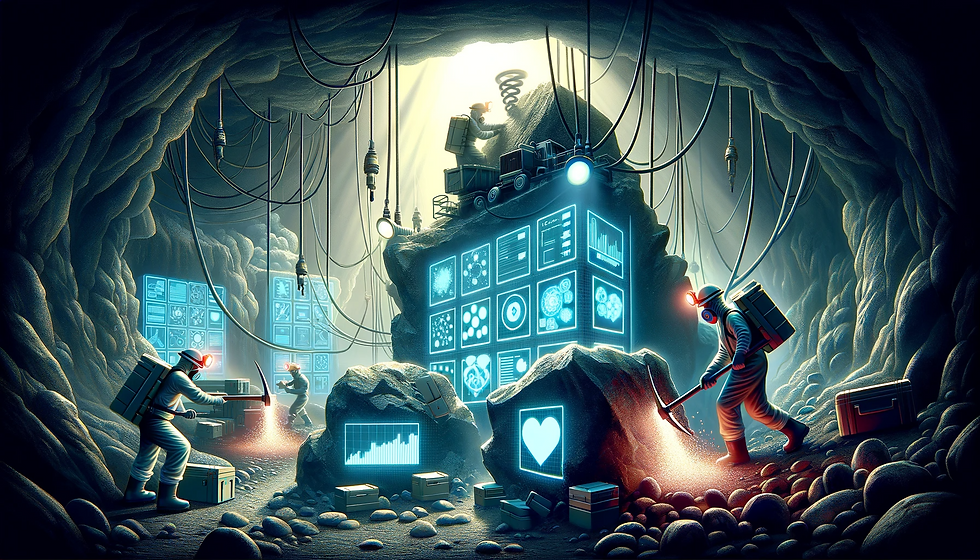
In this article, we'll explore the ways data mining can help figure your way through vast amounts of adverse event information. At each step along the way, I'll add in a code snippet as a basic example of what can be done.
The Wild World of Adverse Event Data
Imagine a dense forest, each tree representing a piece of data. This is the landscape of adverse event reports. It's a vast and complex domain, much like the FDA's MAUDE database, which houses a wealth of information on medical device adverse events. However, this richness comes with challenges, such as inconsistent reporting, incomplete entries, and a diverse range of data formats. Navigating this terrain requires not just technical skill but also a detective's intuition.
Code Snippet - load and view
import pandas as pd
# Load the MAUDE dataset
maude_data = pd.read_csv('MAUDE_data.csv')
# Quick glimpse of the data
print(maude_data.head())
Data Mining: The Art of Finding Patterns
Data mining is the art of uncovering hidden patterns and correlations in large datasets. It involves various techniques, from basic data sorting to complex machine learning algorithms. This process is akin to an archaeologist sifting through soil to find hidden artifacts. In our case, the artifacts are insights that can lead to improved medical device safety and patient care.
Code Snippet - basic cleaning
# Dealing with missing values and duplicates
maude_data = maude_data.drop_duplicates().dropna()
# Lowercasing and stripping text data
maude_data['event_description'] = maude_data['event_description'].str.lower().str.strip()
Taming Messy Data
Data cleaning is an essential first step in any data analysis process. It's the process of transforming raw data into a format that's easier to work with. This involves handling missing values, standardizing text entries, and removing duplicates. Think of it as preparing the room before starting to paint the walls. Without this crucial step, any further analysis might lead to misleading or inaccurate conclusions.
Code Snippet - messy data
# Filling missing values with a placeholder
maude_data['manufacturer'] = maude_data['manufacturer'].fillna('Unknown')
# Standardizing date formats
maude_data['event_date'] = pd.to_datetime(maude_data['event_date'], errors='coerce')
Diving Deeper with Machine Learning
Advancing from basic statistics to machine learning marks a significant step in data analysis. Machine learning models, such as classification and regression algorithms, are powerful tools that can identify complex patterns and relationships in the data. These models are like the supercomputers of our detective agency, processing vast amounts of information to uncover insights that would be impossible to detect manually.
Code Snippet - pattern recognition
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Preparing the dataset for machine learning
X = maude_data[['feature1', 'feature2']] # Example features
y = maude_data['outcome'] # Target variable
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3)
# Training a Random Forest Classifier
model = RandomForestClassifier()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
The Moral Compass
In the realm of data mining, particularly in healthcare, ethical considerations are paramount. We must navigate the delicate balance between extracting valuable insights and respecting individual privacy. Data security, confidentiality, and ethical use of information are not just regulatory requirements but moral imperatives. Missteps in this area can erode public trust and have real-world consequences.
Predicting the Future
The future of data mining in medical device surveillance is not just about understanding the present but predicting the future. Predictive analytics, powered by advanced machine learning models, holds the potential to forecast device malfunctions or adverse events before they occur. This proactive approach could revolutionize medical device safety, turning data analysis from a reactive to a proactive tool in our healthcare arsenal.
Putting It All Together
To demonstrate our data analysis techniques, we need a playground of data. Let's create a synthetic dataset that mimics the structure of real adverse event reports. This dataset will enable us to run our code snippets and provide a hands-on example of how data analysis can be applied in the context of medical device surveillance.
Code Snippet - example data
import numpy as np
# Creating a synthetic dataset
np.random.seed(0)
example_data = pd.DataFrame({
'feature1': np.random.rand(100),
'feature2': np.random.rand(100),
'outcome': np.random.choice(['Malfunction', 'No Issue'], 100)
})
# Displaying the first few rows of the dataset
print(example_data.head())
# Saving the dataset
example_data.to_csv('example_MA_data.csv', index=False)
Now we can run it through our model and start analyzing for insight.
Code Snippet - analysis
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Load the synthetic dataset
data = pd.read_csv('example_MA_data.csv')
# Assuming 'feature1' and 'feature2' might correlate with malfunctions
# Example: Filtering data to focus on potential high-risk cases
high_risk_data = data[data['outcome'] == 'Malfunction']
# Preparing data for analysis
X = high_risk_data[['feature1', 'feature2']] # Features that might indicate risk
y = high_risk_data['outcome'] # Binary outcome: 'Malfunction' or 'No Issue'
# Encoding the 'outcome' column for analysis
y_encoded = y.apply(lambda x: 1 if x == 'Malfunction' else 0)
# Splitting the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y_encoded, test_size=0.3, random_state=42)
# Using a Random Forest Classifier to identify patterns
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Making predictions and evaluating the model
predictions = model.predict(X_test)
# Example evaluation: Print the accuracy of the model
accuracy = model.score(X_test, y_test)
print(f"Model Accuracy: {accuracy * 100:.2f}%")
In this example, we're using a Random Forest Classifier to analyze the synthetic data. We focus on records labeled as 'Malfunction' to understand what features might correlate with these adverse outcomes. This approach mimics real-world analysis, where data scientists seek to identify risk factors or patterns that could lead to device malfunctions.
The Conclusion
As we conclude our journey through the world of medical device post-market surveillance, it's clear that data mining is an invaluable tool in our quest to improve patient safety. Through careful analysis, ethical consideration, and a commitment to continuous learning, we can turn data into actionable insights. In this digital age, our role as data detectives is not just about solving mysteries; it's about making a tangible impact on the lives of patients and the efficacy of medical devices
As always, here's to the vigilance, the innovation, and the commitment that will steer us towards a future where medical devices are safer, more reliable, and more effective than ever before.
If you like (or don't like) what you see, please share, leave a comment, or drop a line on the Contact Page.
Thanks for reading.
Comments